Slide Puzzle Python Code Game
Contents • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • • [ ] We fist define a generic package Generic_Puzzle. Adobe icc profiles linux os. Upon instantiation, it can take any number of rows, any number of columns for a rows*columns-1 game.
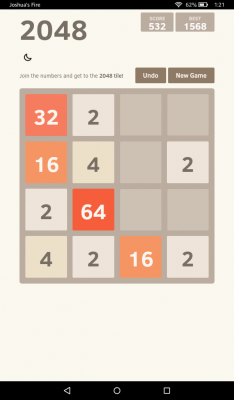
Instead of plain numbers, the tiles on the board can have arbitrary names (but they should all be of the same length). The package user can request the name for the tile at a certain (row,column)-point, and the set of possible moves.
8/15 puzzle using A* (A Star) algorithm. Download 15Puzzle.zip - 16.7 KB; Introduction. Let's talk about 8 puzzle – simple sliding tiles on a 3x3 grid. You are allowed to move along with empty block, one step at a time. And last thing – I didn’t lie when I added 15 puzzle in the list. Same code can be used. In fact, you just need. Using Uninformed & Informed Search Algorithms to Solve 8-Puzzle (n-Puzzle) in Python / Java March 16, 2017 October 28, 2017 / Sandipan Dey This problem appeared as a project in the edX course ColumbiaX: CSMM.101x Artificial Intelligence (AI).
The user can move the empty space up, down, left and right (if possible). If the user makes the attempt to perform an impossible move, a Constraint_Error is raised. Output:./puzzle_15 4 1 2 3 4 5 6 7 8 9 14 10 11 13 15 12 8 Up! 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 6 Right! 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 5 Possible Moves and Commands: u,U,^,8: UP d,D,v,2: DOWN l,L,,6: RIGHT!: Quit 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 6 Right! 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 2 Down!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15! For other puzzles, one must just the single line with the package instantiation. E.g., for an 8-puzzle, we would write the following. Package Puzzle is new Generic_Puzzle (Rows => 3, Cols => 3, Name => Image ); [ ]. Output: prompt$ cobc -xj fifteen.cbl start fifteen puzzle enter a two-digit tile number and press to move press only to exit.. [ ] Credit to this post for help with the inversions-counting function: Run it (after loading the file) with 15 :: main. Output: +--+--+--+--+ 3 7 10 4 +--+--+--+--+ 5 6 11 +--+--+--+--+ 12 8 15 1 +--+--+--+--+ 9 14 13 2 +--+--+--+--+ Choose tile to move: 6 +--+--+--+--+ 3 7 10 4 +--+--+--+--+ 5 6 11 +--+--+--+--+ 12 8 15 1 +--+--+--+--+ 9 14 13 2 +--+--+--+--+ [ ] module Puzzle = struct type t = let make ( ) = [ 15; (* 0: the empty space *) 0; 1; 2; 3; 4; 5; 6; 7; 8; 9; 10; 11; 12; 13; 14; ] let move p n = let hole, i = p.
Omni hotels. ( 0 ) '; Puzzle.move p ( ( ) > ) done To move, input the number to slide into the blank. If you accidentally make an impossible move you can undo it by repeating the last input.
A nice self-checked puzzle, the same as if you were physically moving the pieces around. Output: 1 2 3 4 5 6 8 9 10 7 11 13 14 15 12 1 2 3 4 5 6 8 9 10 7 11 13 14 15 12 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 1 2 3 4 5 6 7 8 9 10 11 13 14 15 12 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 solved! [ ] Console version.
All Puzzles are solvable. #Difficulty is the number of shuffle (default 10). Numbers are displayed in Hexadecimal (ie 1 to F) Default controls are u,d,l,r #difficulty = 10;higher is harder #up = 'u' #down = 'd' #left = 'l' #right = 'r' OpenConsole ( '15 game' ): EnableGraphicalConsole ( 1 ) Global Dim Game.i ( 3, 3 ) Global hole. Point Procedure NewBoard ( ) num.i = 0 For j = 0 To 3 For i = 0 To 3 Game (i,j ) =num num + 1 Next i Next j EndProcedure Procedure.s displayBoard ( ) For j = 0 To 3 For i = 0 To 3 ConsoleLocate (i,j ) If Game (i,j ) = 0: Print ( ' ' ): Continue: EndIf Print ( Hex (Game (i,j ) ) ) Next i Next j PrintN ( ' ) Print ( 'Your Choice Up:' +#up + ', Down:' +#down + ', Left:' +#left + ' Or Right:' +#right + ' ' ) Repeat keypress$ = Inkey ( ) Until keypress$ ' keypress$ = LCase (keypress$ ) ProcedureReturn keypress$ EndProcedure Procedure UpdateBoard (key$ ) If key$ =#up And hole y. Output: 1 2 3 5 7 6 11 13 14 8 4 10 9 15 12 Enter a move: (right left down) right 1 2 3 5 7 6 11 13 14 8 4 10 9 15 12 Enter a move: (left down) down 1 2 3 11 5 7 6 13 14 8 4 10 9 15 12 Enter a move: (left down up) down 1 2 3 11 5 7 6 4 13 14 8 10 9 15 12 Enter a move: (left down up) [ ] tiles=[1:15,0]; solution=[tiles(1:4). Tiles(13:16)]; solution=string(solution); solution(16)=' '; init_pos=grand(1,'prm',tiles); puzzle=[init_pos(1:4).
Init_pos(13:16)]; puzzle=string(puzzle); blank_pos=[]; for i=1:4 for j=1:4 if puzzle(i,j)=='0' then blank_pos=[i,j]; end end end clear i j puzzle(blank_pos(1),blank_pos(2))=' '; n_moves=0; solved=%F; while ~solved disp(puzzle); mprintf(' n'); neighbours=[0 -1. +1 0]; neighbours(:,1)=neighbours(:,1)+blank_pos(1); neighbours(:,2)=neighbours(:,2)+blank_pos(2); neighbours=[neighbours zeros(4,1)] i=0; for i=1:4 if ~(neighbours(i,1)4 . Output: This test was run while making init_pos=[1 2 3 4 5 6 7 8 9 14 10 11 13 0 15 12].!1 2 3 4!!!!5 6 7 8!!!!9 14 10 11!!!!13 15 12! Enter tile you want to move (0 to exit): 14!1 2 3 4!!!!5 6 7 8!!!!9 10 11!!!!13 14 15 12! Enter tile you want to move (0 to exit): 10!1 2 3 4!!!!5 6 7 8!!!!9 10 11!!!!13 14 15 12!